Dictionaries in Python Key Value Pairs Methods and Iteration
13 February 2025
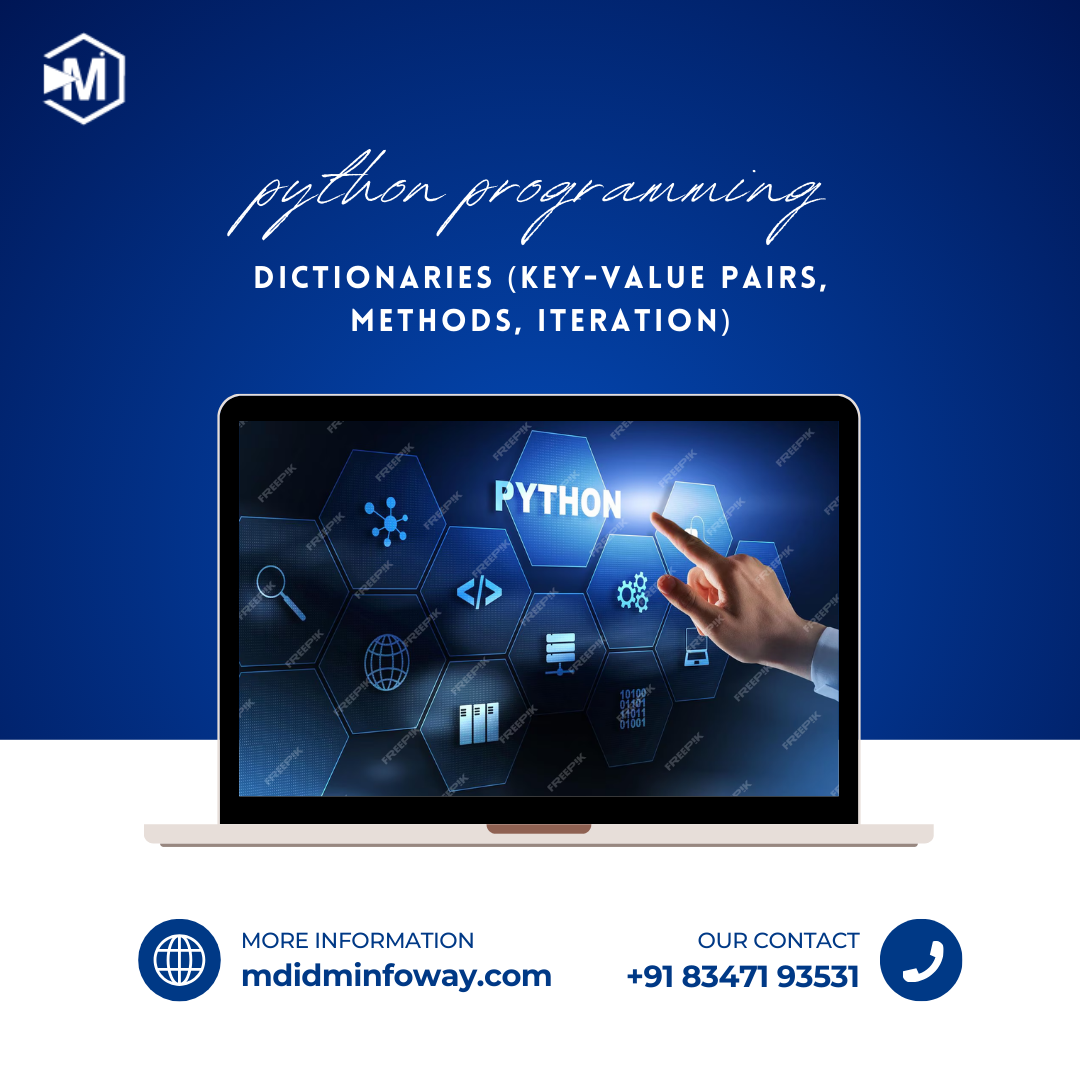
Dictionaries in Python: Key-Value Pairs, Methods, and Iteration
A dictionary in Python ia a collection of key-value pairs that allow efficient data retrieval and manipulation. Unlike lists and tuples, dictionaries are unordered (before Python 3.7) and matable collections, making them ideal for mapping relationships between data.
Key Features of Dictionaries
- Key-Value Pair Structure: Each item consists of a unique key mapped to a corresponding value.
- Fast Lookup: Optimized for retrieving values based on keys.
- Mutable: Can be modified after creation (keys must be immutable, but values can be changed).
- Unordered (Before Python 3.7): From Python 3.7 onwards, dictionaries maintain insertion oeder.
Creating a Dictionary
Dictionaries are defined using curly braces {} with key-value pairs separated by colons (:).
# Example of a dictionary
student = {
"name": "Alice",
"age": 22,
"grade": "A"
}
print(student)
# Output: {'name': 'Alice', 'age': 22, 'grade': 'A'}
Alternatively, use the dict() constructor:
student = dict(name="Alice", age=22, grade="A")
Accessing Dictionary Elements
Values are accessed using keys:
print(student["name"]) # Output: Alice
Using .get() prevents errors if a key doesn't exist:
print(student.get("course", "Not Assigned"))
# Output: Not Assigned (instead of an error)
Modifying a Dictionary
Adding a new key-value pair:
student["course"] = "Computer Science"
Updating an existing value:
student["grade"] = "A+"
Removing a key-value pair:
student.pop("age") # Removes 'age' key
Dictionary Methods
Python provides useful methods for dictionary manipulation:
print(student.keys()) # Output: dict_keys(['name', 'grade', 'course'])
print(student.values()) # Output: dict_values(['Alice', 'A+', 'Computer Science'])
print(student.items()) # Output: dict_items([('name', 'Alice'), ('grade', 'A+'), ('course', 'Computer Science')])
To remove all items:
student.clear()
print(student) # Output: {}
Iterating Over a Dictionary
Dictionaries can be looped through using for loops:
for key, value in student.items():
print(f"{key}: {value}")
Output:
name: Alice
grade: A+
course: Computer Science
Dictionary Use Cases
- Storing Configuration Settings
- Mapping Relationships (e.g., Employee ID to Name)
- Efficiently Storing and Retrieving Data
Dictionary vs. List : Key Differences
Feature : Access Speed
Dictionary : Faster (Key Lookup)
List : Slower (Index-based)
Feature : Structure
Dictionary : Key-Value Pairs
List : Indexed Elements
Feature : Order (Pre-3.7)
Dictionary : Unordered
List : Ordered
Feature : Mutability
Dictionary : Mutable
List : Mutable
Dictionaries are powerful for structured data storage, making them an essential tool in Python programming.