Defining and Calling Functions in Python
13 February 2025
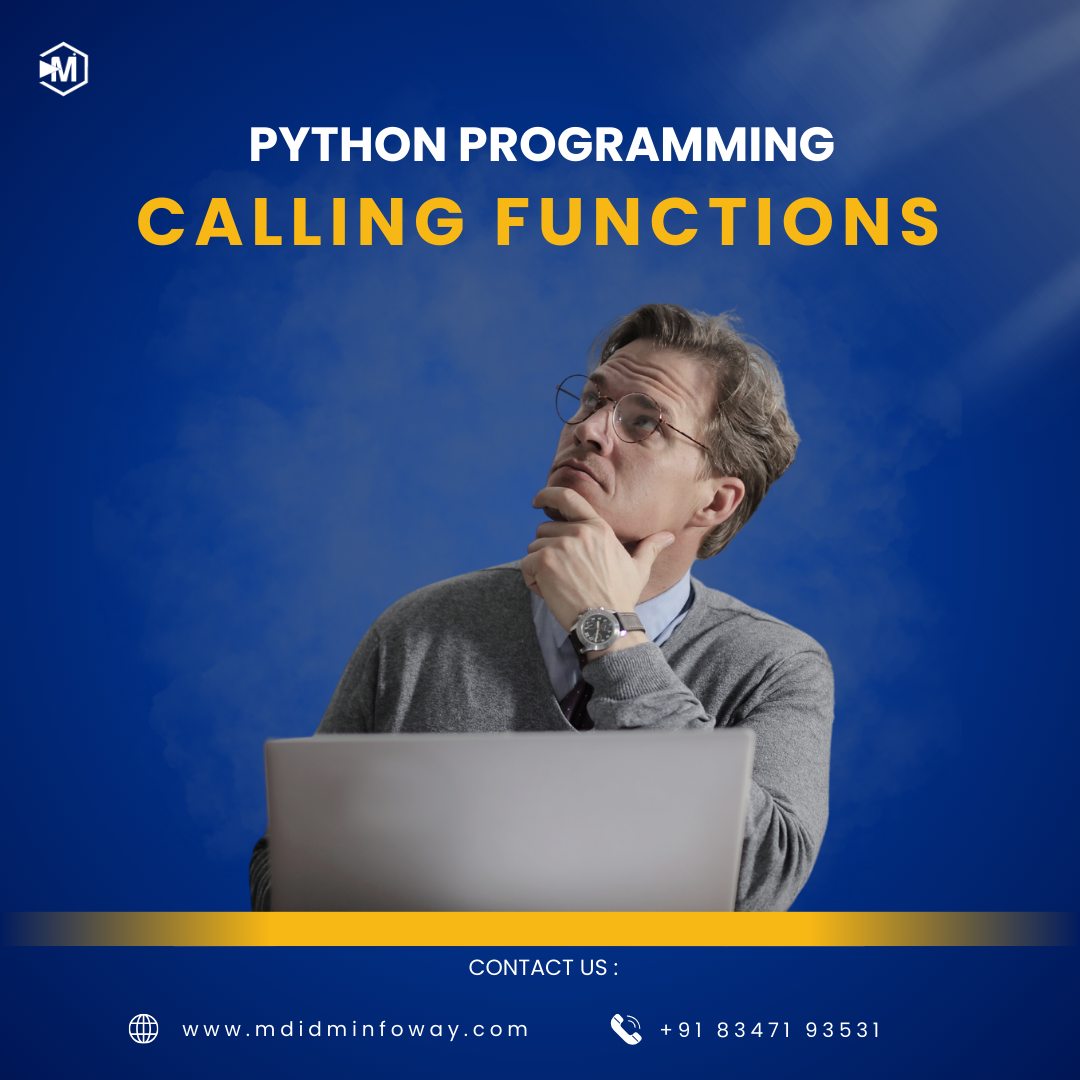
Defining and Calling Functions in Python
Functions are reusable blocks of code that perform a specific task. They help in organizing code, improving readability, and reducing redundancy.
1. Defining a Functions:
A function is defined using the def keyword, followed by a function name and parentheses. It can take parameters and return a value.
Syntax:
def function_name(parameters):
"""Optional docstring describing the function"""
# Function body
return value # (optional)
2. Calling a Function:
A function is called by using its name followed by parentheses. If the function accepts arguments, values must be passed inside the parentheses.
Syntax:
function_name(arguments)
Examples of Function Usage
Example 1 : Function Without Parameters
def greet():
print("Hello! Welcome to Python functions.")
# Calling the function
greet()
Output:
Hello! Welcome to Python functions.
Example 2 : Function with Parameters
def add_numbers(a, b):
return a + b
# Calling the function with arguments
result = add_numbers(5, 3)
print("Sum:", result)
Output:
Sum: 8
Example 3 : Function with Default Parameters
def greet(name="Guest"):
print(f"Hello, {name}!")
# Calling the function without an argument
greet()
# Calling the function with an argument
greet("Alice")
Output:
Hello, Guest!
Hello, Alice!
Example 4 : Function Returning Multiple Values
def calculate(a, b):
sum_result = a + b
diff_result = a - b
return sum_result, diff_result
# Calling the function
sum_val, diff_val = calculate(10, 4)
print("Sum:", sum_val)
print("Difference:", diff_val)
Output:
Sum: 14
Difference: 6
Key Benefits of Using Functions
- Code Reusability - Write once, use multiple times.
- Modularity - Breaks complex problems into smaller functions.
- Improved Readability - Organized and structured code.
- Easier Debugging - Isolate issues in specific functions.
Functions are fundamental in Python programming, allowing for effcient and scalable code development.